Write a Few Lines of Code to Control a Smart Device!
Mature design solutions and standard production processes provide developers with stable and reliable products and services, focusing on business development;
Rich product series and comprehensive equipment functions reduce equipment self-development time and technical risks for customers, and save project costs.
Rich product series and comprehensive equipment functions reduce equipment self-development time and technical risks for customers, and save project costs.
MemoryPersistence persistence = new MemoryPersistence();
MqttConnectOptions connOpts = new MqttConnectOptions();
MqttClient client = new MqttClient(broker, clientId, persistence);
client.connect(connOpts);
MqttMessage message = new MqttMessage(content.getBytes());
message.setQos(qos);
client.publish(pub_topic, message);
System.out.println('Message published');
client.subscribe(sub_topic, qos);
System.out.println('Subscribed to topic: ' + sub_topic);
System.out.println('Subscribed to message: ' + message);
MqttConnectOptions connOpts = new MqttConnectOptions();
MqttClient client = new MqttClient(broker, clientId, persistence);
client.connect(connOpts);
MqttMessage message = new MqttMessage(content.getBytes());
message.setQos(qos);
client.publish(pub_topic, message);
System.out.println('Message published');
client.subscribe(sub_topic, qos);
System.out.println('Subscribed to topic: ' + sub_topic);
System.out.println('Subscribed to message: ' + message);
void publish(MQTTClient client, char *topic, char *payload) {
MQTTClient_message message = MQTTClient_message_initializer;
message.payload = payload;
message.payloadlen = strlen(payload);
message.qos = QOS;
message.retained = 0;
MQTTClient_deliveryToken token;
MQTTClient_publishMessage(client, topic, &message, &token);
MQTTClient_waitForCompletion(client, token, TIMEOUT);
printf('Send `%s` to topic `%s` ', payload, PUB_TOPIC);
}
MQTTClient_message message = MQTTClient_message_initializer;
message.payload = payload;
message.payloadlen = strlen(payload);
message.qos = QOS;
message.retained = 0;
MQTTClient_deliveryToken token;
MQTTClient_publishMessage(client, topic, &message, &token);
MQTTClient_waitForCompletion(client, token, TIMEOUT);
printf('Send `%s` to topic `%s` ', payload, PUB_TOPIC);
}
def publish(client):
msg_count = 0
while not FLAG_EXIT:
msg_dict = {
'type': 'info'
}
msg = json.dumps(msg_dict)
if not client.is_connected():
logging.error('publish: MQTT client is not connected!')
time.sleep(1)
continue
result = client.publish(PUB_TOPIC, msg)
status = result[0]
if status == 0:
print(f'Send `{msg}` to topic `{PUB_TOPIC}`')
else:
print(f'Failed to send message to topic {PUB_TOPIC}')
msg_count += 1
time.sleep(1)
msg_count = 0
while not FLAG_EXIT:
msg_dict = {
'type': 'info'
}
msg = json.dumps(msg_dict)
if not client.is_connected():
logging.error('publish: MQTT client is not connected!')
time.sleep(1)
continue
result = client.publish(PUB_TOPIC, msg)
status = result[0]
if status == 0:
print(f'Send `{msg}` to topic `{PUB_TOPIC}`')
else:
print(f'Failed to send message to topic {PUB_TOPIC}')
msg_count += 1
time.sleep(1)
func publish(client mqtt.Client) {
qos := 0
msgCount := 0
for {
payload := `{'type':'info'}`
if token := client.Publish(pub_topic, byte(qos), false, payload); token.Wait() && token.Error() != nil {
fmt.Printf('publish failed, topic: %s, payload: %s', pub_topic, payload)
} else {
fmt.Printf('publish success, topic: %s, payload: %s', pub_topic, payload)
}
msgCount++
time.Sleep(time.Second * 1)
}
}
qos := 0
msgCount := 0
for {
payload := `{'type':'info'}`
if token := client.Publish(pub_topic, byte(qos), false, payload); token.Wait() && token.Error() != nil {
fmt.Printf('publish failed, topic: %s, payload: %s', pub_topic, payload)
} else {
fmt.Printf('publish success, topic: %s, payload: %s', pub_topic, payload)
}
msgCount++
time.Sleep(time.Second * 1)
}
}
client.on('connect', () => {
console.log(`${protocol}: Connected`)
client.subscribe(sub_topic, { qos }, (error) => {
if (error) {
console.log('subscribe error:', error)
return
}
console.log(`${protocol}: Subscribe to topic '${sub_topic}'`)
client.publish(pub_topic, payload, { qos }, (error) => {
if (error) {
console.error(error)
}
})
})
})
console.log(`${protocol}: Connected`)
client.subscribe(sub_topic, { qos }, (error) => {
if (error) {
console.log('subscribe error:', error)
return
}
console.log(`${protocol}: Subscribe to topic '${sub_topic}'`)
client.publish(pub_topic, payload, { qos }, (error) => {
if (error) {
console.error(error)
}
})
})
})
static void Publish(MqttClient client, string topic)
{
int msg_count = 0;
while (true)
{
System.Threading.Thread.Sleep(1*1000);
string msg = '{'type':'info'}';
client.Publish(topic, System.Text.Encoding.UTF8.GetBytes(msg));
Console.WriteLine('Send `{0}` to topic `{1}`', msg, topic);
msg_count++;
}
}
{
int msg_count = 0;
while (true)
{
System.Threading.Thread.Sleep(1*1000);
string msg = '{'type':'info'}';
client.Publish(topic, System.Text.Encoding.UTF8.GetBytes(msg));
Console.WriteLine('Send `{0}` to topic `{1}`', msg, topic);
msg_count++;
}
}
$payload = array(
'type' => 'info'
);
$jsonp = json_encode($payload);
$mqtt->publish(
$jsonp,
0,
true
);
printf('msg send $jsonp to $pub_topic');
$mqtt->subscribe($sub_topic, function ($topic, $message) {
printf('Received message on topic [%s]: %s', $topic, $message);
}, 0);
'type' => 'info'
);
$jsonp = json_encode($payload);
$mqtt->publish(
$jsonp,
0,
true
);
printf('msg send $jsonp to $pub_topic');
$mqtt->subscribe($sub_topic, function ($topic, $message) {
printf('Received message on topic [%s]: %s', $topic, $message);
}, 0);
For more MQTT example code, please refer to best practices
Focusing on Software Developers
Provide Useful IoT Smart Devices
Provide Useful IoT Smart Devices
In 2022, the School of Computer and Artificial Intelligence at Wuhan University of Technology established an intelligent device development and software development team,
After nearly two years of exploration and optimization, GeekOpen has been launched ™ Commercial series intelligent devices,
As of the end of 2023, the equipment has been installed and served on over 500 commercial projects, covering more than 10 countries and regions worldwide!
After nearly two years of exploration and optimization, GeekOpen has been launched ™ Commercial series intelligent devices,
As of the end of 2023, the equipment has been installed and served on over 500 commercial projects, covering more than 10 countries and regions worldwide!

Open Device Functionality
GeekOpen ™ Open all functions of the device without reservation, created by software developers to meet the needs of more IoT scenarios and unleash more IoT capabilities.

Unified Protocol
GeekOpen offers design solutions for different chips ™ Through extensive efforts, it is still possible to form a unified communication protocol that fully supports MQTT and TCP protocols.

Global Device Version
For each device, GeekOpen ™ We will design an adaptive version according to the specifications and requirements of different regions around the world, and design corresponding molds for assembly and reproduction.

OEM/ODM
When the purchase of equipment reaches 1000 units per batch, OEM can be carried out according to customer requirements. For customers who require ODM, please contact GeekOpen for detailed requirement communication.
The Device Supports Wifi Or 4G Full Network Connectivity,
Connecting To Network Services
Connecting To Network Services
GeekOpen ™ The WiFi communication range of the device is generally 10-50 meters, and it can connect to the customer's local or cloud services
The 4G network supports three networks: mobile, telecommunications, and China Unicom.
It is suitable for sites with large equipment distribution distances or unsuitable for network construction, and can only connect to customer cloud services.
The 4G network supports three networks: mobile, telecommunications, and China Unicom.
It is suitable for sites with large equipment distribution distances or unsuitable for network construction, and can only connect to customer cloud services.
Wifi Connection:
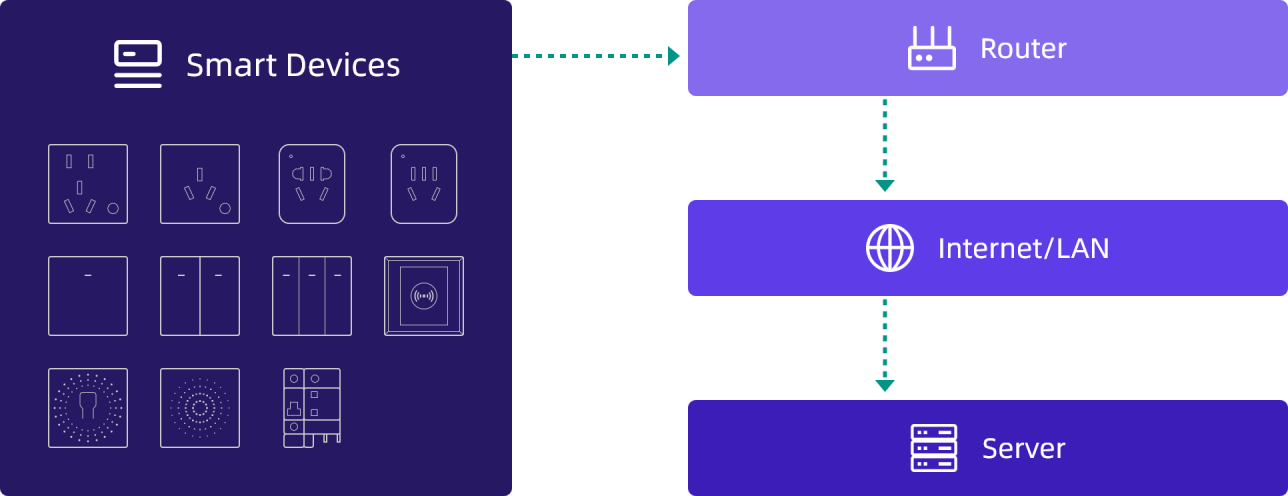
Using WiFi smart devices, users can deploy servers on their local LAN or cloud. The device uses the MQTT protocol to exchange data with the server through subscription or publication, or uses the TCP protocol to exchange data with the server through listening, suitable for scenarios where the device is deployed centrally.
4G Full Network Connection:
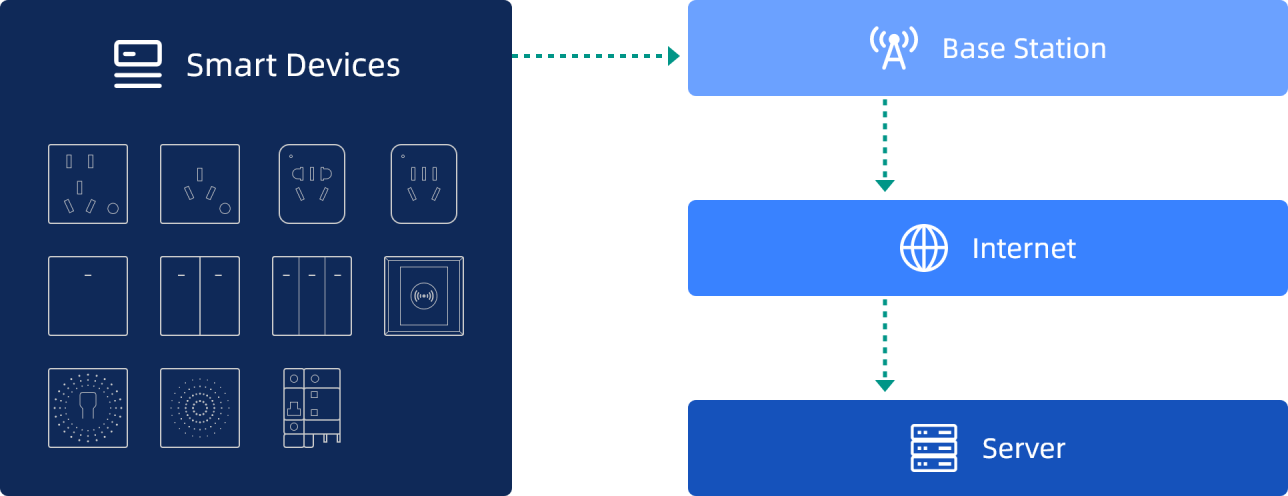
Using 4G smart devices, users can only deploy servers in the cloud. The device uses the MQTT protocol to exchange data with the server through subscription or publication, or uses the TCP protocol to exchange data with the server through listening, which is suitable for scenarios where the device deployment is more dispersed.
Use MQTT Or TCP Protocol To Maintain
Device Communication
Device Communication
Support customers to build their own MQTT services, publish or subscribe to device messages based on message queues, for controlling devices or collecting device data.
Customers can also set up devices to connect to TCP Server services, which is suitable for traditional device communication, such as using Netty to create TCP Server or directly connecting devices using the upper computer.
We have listed the following three commonly used device networking communication methods for customer project reference.
Customers can also set up devices to connect to TCP Server services, which is suitable for traditional device communication, such as using Netty to create TCP Server or directly connecting devices using the upper computer.
We have listed the following three commonly used device networking communication methods for customer project reference.
Based On MQTT Server
Communication:
Communication:
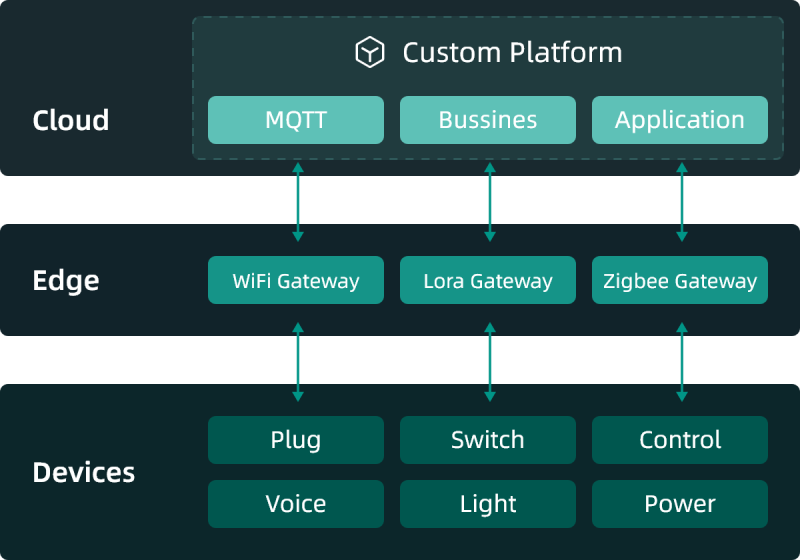
Connect to GeekOpen™ in a reliable, efficient, and secure manner through the standard MQTT protocol Smart devices enable your IoT business
Based On TCP Server
Communication:
Communication:
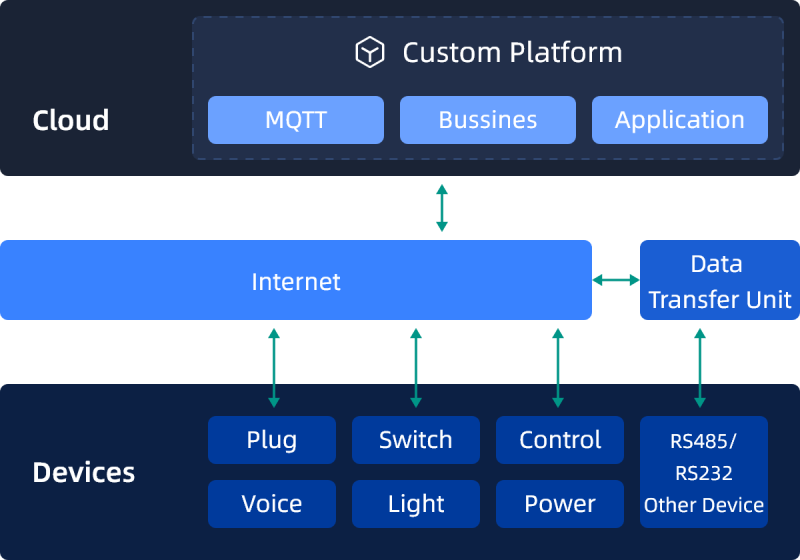
The customer customizes the TCP Server and uses the TCP protocol to connect to the device. It can directly use the existing network to parallel with other devices and achieve application expansion.
Based On Upper Computer
Communication:
Communication:
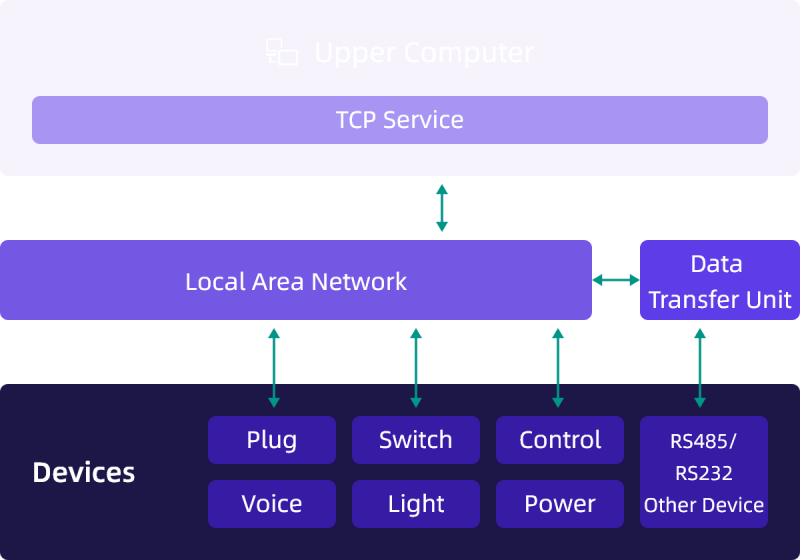
Customers can also configure GeekOpen™ The intelligent device TCP service upper computer directly collects data and controls the device through configuration tools.
Stable Basic Functions and Free Implementation
of Business Development
GeekOpen ™ Complex hardware instructions have been repackaged into software messages using standard JSON format.
After connecting the device to MQTT services, data collection and control can be achieved through simple message publishing or subscription.
Both front-end and back-end developers can quickly take over devices and achieve personalized application development.
After connecting the device to MQTT services, data collection and control can be achieved through simple message publishing or subscription.
Both front-end and back-end developers can quickly take over devices and achieve personalized application development.

Power On/Off
MQTT publishes topic messages, or TCP Server sends messages to the Client
Send json messages(Power ON)
{
"type":"event",
"key":1
}
"type":"event",
"key":1
}
Return json messages
{
"type":"Socket-mini",
"key":1,
"wifiLock":0,
"keyLock":0,
"ip":"192.168.0.101"
}
"type":"Socket-mini",
"key":1,
"wifiLock":0,
"keyLock":0,
"ip":"192.168.0.101"
}

Electricity inquiry
Query real-time voltage, current, and cumulative power consumption for monitoring equipment load and power consumption process
Send json messages
{
"type":"statistic"
}
"type":"statistic"
}
Return json messages
{
"voltage": 226.024,
"current": 15.027,
"power": 2921.511,
"energy": 25.047
}
"voltage": 226.024,
"current": 15.027,
"power": 2921.511,
"energy": 25.047
}

WiFi Lock
It is used to lock the WiFi information of the device, which will not be misoperated by on-site personnel and ensure the correct WiFi information
Send json messages
{
"type":"setting",
"wifiLock":1
}
"type":"setting",
"wifiLock":1
}
Return json messages
{
"type":"Socket-mini",
"key":1,
"wifiLock":1,
"keyLock":0,
"ip":"192.168.0.101"
}
"type":"Socket-mini",
"key":1,
"wifiLock":1,
"keyLock":0,
"ip":"192.168.0.101"
}

Function KeyLock
Used to lock device function buttons and prevent on-site personnel from long pressing to reset the device
Send json messages
{
"type":"setting",
"keyLock":1
}
"type":"setting",
"keyLock":1
}
Return json messages
{
"type":"Socket-mini",
"key":1,
"wifiLock":1,
"keyLock":1,
"ip":"192.168.0.101"
}
"type":"Socket-mini",
"key":1,
"wifiLock":1,
"keyLock":1,
"ip":"192.168.0.101"
}

Status Query
The current working status of the device, including the MAC address and WiFi name
Send json messages
{
"type":"info"
}
"type":"info"
}
Return json messages
{
"mac":"Socket-mini",
"type":"Socket-mini",
"version":"2.0.0",
"key":1,
"wifiLock":1,
"keyLock":1,
"ip":"192.168.0.101",
"ssid":"open-wifi"
}
"mac":"Socket-mini",
"type":"Socket-mini",
"version":"2.0.0",
"key":1,
"wifiLock":1,
"keyLock":1,
"ip":"192.168.0.101",
"ssid":"open-wifi"
}
Support Global Developers to Provide
AC100v-250v Wide Voltage Devices
Suitable for multiple scenarios, freely combined and fully controlled, easy to develop software to control GeekOpen intelligent hardware,
JSON data transmission, response speed less than 50 milliseconds, supporting multilingual development
JSON data transmission, response speed less than 50 milliseconds, supporting multilingual development
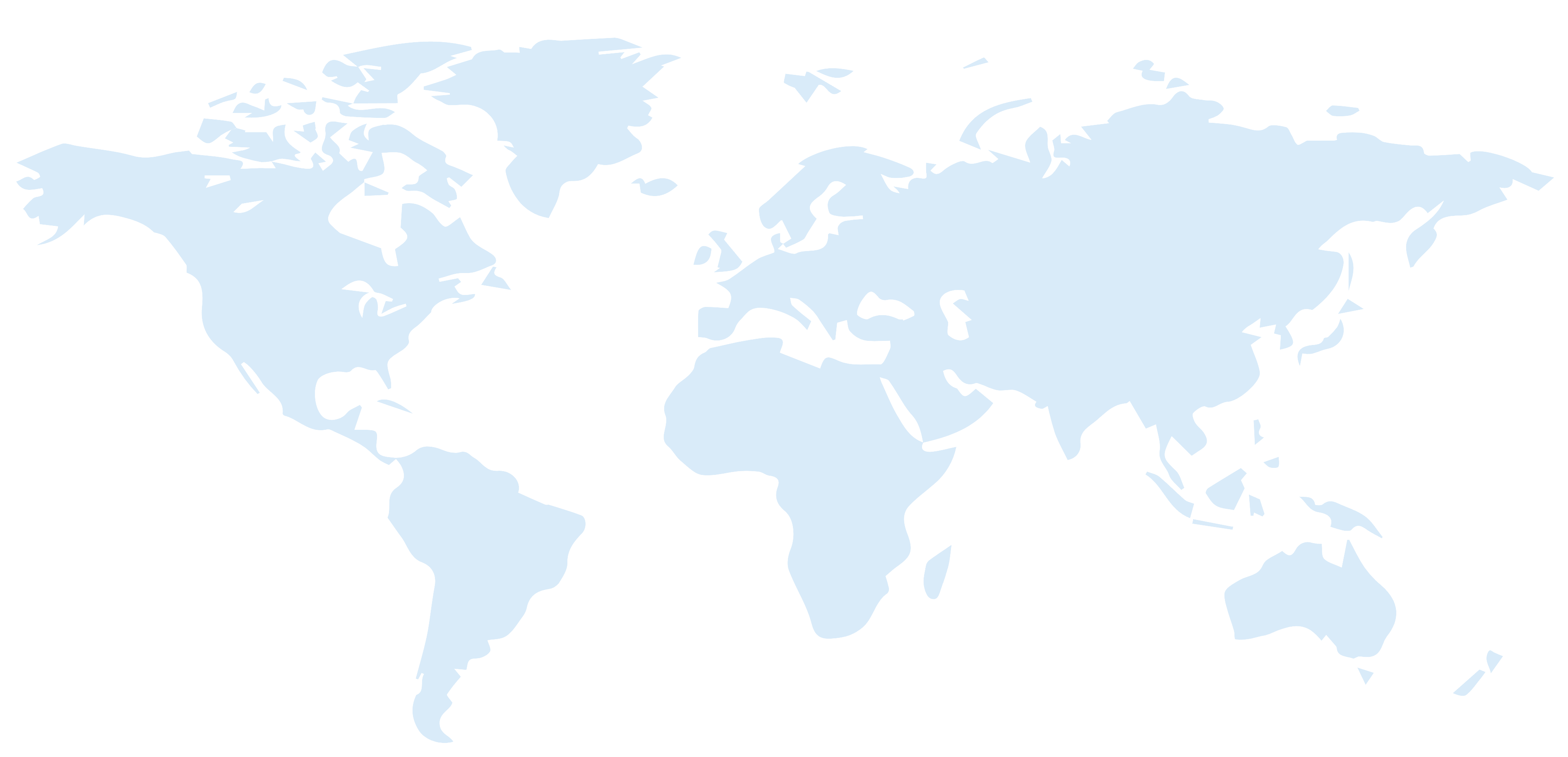
US Standard Adaptation
Taiwan, the United States, Canada - the Americas, Mexico - the Americas, Japan - Asia, Brazil - the Americas, Philippines - Asia, Thailand - Asia, Lebanon - Asia, Venezuela - the Americas, Panama - the Americas, Colombia - the Americas, Ecuador - the Americas, Laos - Asia, etc.
Australian Standard Adaptation
China Mainland, Australia - Oceania, New Zealand - Oceania, Fiji - Oceania, Norway - Europe, Turkey - Asia/Europe, Iran - Asia, Israel - Asia, Jordan - Asia, Kuwait - Asia, Argentina - America, etc.
British Standard Adaptation
Hong Kong, Macau, United Kingdom - Europe, India - Asia, Pakistan - Asia, Singapore - Asia, Malaysia - Asia, Vietnam - Asia, Zimbabwe - Africa, Indonesia - Asia, Maldives - Asia, Qatar - Asia, Arab - Asia, etc.